GEO Pay API
API Endpoint
https://partners.geo-pay.net/api/v3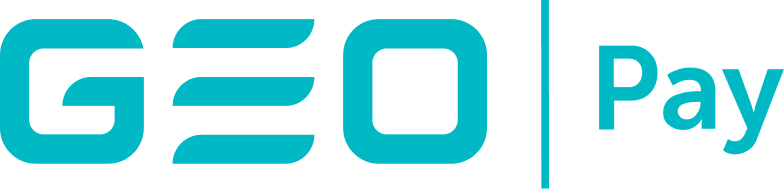
Overview ¶
Authorization
All requests to API services are made through https requests to partners.geo-pay.net/api/. Each request must contain a required parameter - an API key, which uniquely identifies the partner company. API_KEY is issued by us for the partner company.
Security
GEO-Pay services use 256bit https - an end-to-end traffic encryption mechanism, allowing to protect all outgoing and incoming traffic from being monitored / modified by a third party.
Each request and response contains a signature that is generated using the ecdsa algorithm or via the alternative method openssl (for partners with source code in JS or PHP).
KEY GENERATION (ECDSA)
To create a signature, you first need to generate a key pair in the format P521 / secp521r1.
Key generation example using openssl.
Command to generate a private key:
openssl ecparam -name secp521r1 -genkey -noout -out PATH_TO_PRIVATE_KEY
Command to get a public key from a private one:
openssl ec -in PATH_TO_PRIVATE_KEY -pubout -out PATH_TO_PUBLIC_KEY
Next, you give us your public key, then we generate our keys and give you our public key so that you can verify the signature of the responses with it. After that, you will need to sign each request.
KEY GENERATION (OPENSSL)
Command to generate a private key:
openssl genrsa -out key.pem 1024
Command to get a public key from a private one:
openssl rsa -in key.pem -outform PEM -pubout -out public.pem
REQUEST SIGNATURE (ECDSA)
For POST requests, as data for the signature, we use the request parameters in the json format (in the examples, everything in data
), and the signature itself is passed in the request body with the sig
parameter.
When generating the signature, we use the sha256 hash function. We transfer the signature in hex format.
An example of generating a signature in Python:
import ecdsa
import hashlib
sk = ecdsa.SigningKey.from_pem(privatefile.read(), hashfunc=hashlib.sha256)
sig = sk.sign(data.encode(), hashfunc=hashlib.sha256)
return sig.hex()
REQUEST SIGNATURE (OPENSSL)
An example of generating a signature in Python:
import base64
from OpenSSL import crypto
prkey = crypto.load_privatekey(crypto.FILETYPE_PEM, privatefile.read())
signature = crypto.sign(prkey, data.encode(), 'sha256')
signature = base64.b64encode(signature)
RESPONSE SIGNATURE VERIFICATION (ECDSA)
To verify the signature, we use the data response field (all response data in this field in json format) as data for the signature, the signature itself is indicated in the sig response field. Similar to the generation, the signature is in hex format, for verification you need to use the sha256 hash function.
An example of generating a signature in Python:
import ecdsa
import hashlib
vk = ecdsa.VerifyingKey.from_pem(self.public_key)
valid = vk.verify(bytes.fromhex(signature), data.encode('utf-8'), hashfunc=hashlib.sha256)
return valid
RESPONSE SIGNATURE VERIFICATION (OPENSSL)
An example of generating a signature in Python:
import base64
from OpenSSL import crypto
public_key = crypto.load_publickey(crypto.FILETYPE_PEM, file_public_key)
cert = crypto.X509()
cert.set_pubkey(public_key)
try:
signature = base64.b64decode(signature)
crypto.verify(cert, signature, data.encode(), 'sha256')
valid = True
except Exception:
valid = False
Replay attack
The SSLv3 standard protects traffic from replay attacks by means of a monotonically increasing message counter used in a data session. In most cases, this mechanism reliably protects against the attack of a repeated message (request), but it should be borne in mind that a request to carry out one or another operation can be intercepted and re-executed at the OS level, firewall, or any other system that has a priority access to network traffic.
API Payment ¶
Payments. Perform payment ¶
Headers
Content-Type: application/json
Body
{
"data": "{
"api_key": "APIKEY0367c1943...eaf54",
"equivalent": "ushg",
"receiver_hash_id": "45dcdf0c99e0...01d533f93a8b445cf27",
"amount": "150",
"reason": "some text",
"pay_over": false,
"partner_transaction_id": "555bb23...ade3",
}",
"sig": "01fc...61e"
}
Headers
Content-Type: application/json
Body
{
"code": 0,
"message": "OK",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 21,
"message": "Something went wrong",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 23,
"message": "Equivalent is locked for this user.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 1,
"message": "Some parameters are absent, or invalid.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 3,
"message": "Receiver can't be equal to sender.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 13,
"message": "IP address not registered",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 6,
"message": Duplicated value for unique field 'partner_transaction_id'",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Perform paymentPOST/payments/
IMPORTANT! data
field is passed not as an object, but as a JSON string
- data
string
(required)JSON string
- api_key
string
(required)API KEY
- equivalent
string
(required)uahg, kztg, inrg
- receiver_hash_id
string
(required)hash_id user GEO pay
- amount
string
(required)amount
- reason
string
(optional)comment that will be displayed in the payment history
- partner_transaction_id
string
(required)id partner transactions
- sig
string
(required)signature
Payments. Payment status ¶
Headers
Content-Type: application/json
Body
{
"data": "{
"api_key":"APIKEY0367c1943...eaf54",
"transaction_uuid": "654srg...56dfg",
"partner_transaction_id": "555bb23...ade3"
}",
"sig":"01fc...61e"
}
Headers
Content-Type: application/json
Body
{
"code": 0,
"message": "OK",
"data": "{
"uuid": "33f3b592-60ad-4c9e-80d3-5b7b96577df8",
"partner_transaction_id": "555bb23...ade3",
"status": "DONE",
"status_code": "0",
"start_date": "2019-07-08T12:58:18.223397+00:00",
"amount": "100",
"equivalent": "uahg"
}",
"sig": "0049d...2664a"
}
possible statuses:
"DONE", "PENDING", "REJECTED"
Headers
Content-Type: application/json
Body
{
"code": 1,
"message": "Some parameters are absent, or invalid.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 13,
"message": "IP address not registered",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 22,
"message": "There is no transaction with such id",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 23,
"message": "Invalid transaction_id: partner_transaction_id required.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Payment statusPOST/payments/status/
Parameters transaction_uuid
and partner_transaction_id
are optionals
To obtain a status, any one can be specified, but one of them must be specified.
IMPORTANT! data
field is passed not as an object, but as a JSON string
- data
string
(required)JSON string
- api_key
string
(required)API KEY
- transaction_uuid
string
(optional)id transactions GEO pay
- partner_transaction_id
string
(optional)id partner transactions
- sig
string
(required)signature
API Invoices ¶
Callback_url notifications for invoices
For each partner, you can specify a callback_url by contacting support. If it is specified, when the user pays for an invoice, a POST request with transaction data will be made to this callback_url. If status code 200 was not received in response, then it will be repeated with increasing intervals. Maximum retries - 120.
Example request body:
{
"status": "EXPIRED",
"start_date": "2020-08-20T06:33:01.946365+00:00",
"user_hash_id": "059210f3ebfef5f...85113466043c",
"amount": "34.30",
"clear_amount": "31.05",
"transaction_id": "a22c04cc...sd32v151cefd2ac",
"transaction_uuid" : "f5ffea1ae7c5...5ccd2a23969b11334",
"user_hash_id": "1h2l3...qwe4gu",
"partner_transaction_id": "555bb23...ade3"
}
Important: Notifications are not protected or checked for data integrity. If you received a notification, then you should not immediately make a deposit / withdrawal of funds, you need to double-check the payment status via API.
Important: Upon successful payment of the invoice, the user will be redirected to the url specified by you in the link for payment of the invoice. In the parameters of the redirect link, we indicate the status of the payment, but in order to avoid unforeseen situations, it is very important not to credit the user with funds until the status of the payment is checked by API!
Invoice. Create invoice ¶
To test the integration when verifying a payment, we recommend creating another test account, rather than making a payment from the merchant’s account.
Since when making a payment from one account to the same, the payment will be automatically declined.
Headers
Content-Type: application/json
Body
{
"data": "{
"api_key": "APIKEY0367c1943...eaf54",
"equivalent": "uahg",
"amount": "100",
"receiver_hash_id": "45dcdf0c99e0...01d533f93a8b445cf27",
"receiver_phone_number": "380XXXXXXXXX",
"reason": "some text",
"redirect_url": null,
"callback_url": null,
"partner_transaction_id": "555bb23...ade3",
"payment_method": "p2pay" or "geo_pay",
"card_number": "4444333322221111",
"bank_name": "mono_bank"
}",
"sig": "01fc...61e"
}
Headers
Content-Type: application/json
Body
{
"code": 0,
"message": "OK",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00",
"invoice": {
"uuid": "abcabc1f-3333-2222-1111-0f2df6e6a2ed",
"qr_code": "data:image/png;base64",
"url": ""
}
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 20,
"message": "Equivalent not found.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 1,
"message": "Some parameters are absent, or invalid.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 13,
"message": "IP address not registered",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Create invoicePOST/invoices/
IMPORTANT! data
field is passed not as an object, but as a JSON string
- data
string
(required)JSON string
- api_key
string
(required)API KEY
- equivalent
string
(required)uahg, kztg, inrg
- amount
string
(required)сума
- receiver_hash_id
string
(required)hash_id user GEO pay
- receiver_phone_number
string
(optional)phone user GEO pay (required for special settings)
- reason
string
(optional)comment that will be displayed in the payment history
- redirect_url
string
(optional)redirect link after payment
- callback_url
string
(optional)link for callback
- partner_transaction_id
string
(required)id partner transactions
- payment_method
string
(optional)choice of payment method by card or GEO Wallet
- external_account_id
string
(optional)unique identifier of the exchange user who initiated the request to create an invoice
- card_number
string
(optional)user’s card from which they will make a transfer
- bank_name
string
(optional)bank name on the card in Latin script
Examples: (mono_bank, privat_bank, a_bank, sense_bank, kredo_bank, otp_bank, oschad_bank, pivdennyi_bank, fuib_bank, raiffeisen_bank, tascom_bank, ukrgas_bank, ukrexim_bank, ukrsib_bank, universal_bank, credit_agricole_bank, other)- sig
string
(required)signature
Invoice. Invoice status ¶
Headers
Content-Type: application/json
Body
{
"data": "{
"api_key":"APIKEY0367c1943...eaf54",
"transaction_uuid":"654srg...56dfg",
"partner_transaction_id": "555bb23...ade3"
}",
"sig": "00974e2.....3011"
}
Headers
Content-Type: application/json
Body
{
"code": 0,
"message": "OK",
"data": "{
"transaction_id": "039652e4-f619-47a9-b979-609df4328a48",
"redirect_url": null,
"partner_transaction_id": null,
"status": "EXPIRED",
"details": null,
"amount": "112.00000000",
"used": false,
"reason": "some text",
"card_mask": "1111********4444",
"equivalent": {
"type": "fiat",
"tickers": ["uahg", "grn"],
"decimal_places": 2,
"title": "UAH"
},
"receiver": {
"hash_id": "1h2l3...qwe4gu"
},
"datetime": "2021-07-27T16:04:05.355528+00:00"
}",
"sig": "010b63b...efc"
}
possible statuses :
"PROCESSING", "PAID", "REJECTED", "EXPIRED"
Headers
Content-Type: application/json
Body
{
"code": 1,
"message": "Some parameters are absent, or invalid.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 13,
"message": "IP address not registered",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 1,
"message": "There is no invoice with such id.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Invoice statusPOST/invoices/status/
Parameters transaction_uuid
and partner_transaction_id
are optionals
To obtain a status, any one can be specified, but one of them must be specified.
IMPORTANT! data
field is passed not as an object, but as a JSON string
- data
string
(required)JSON string
- api_key
string
(required)API KEY
- transaction_uuid
string
(optional)id transactions GEO pay
- partner_transaction_id
string
(optional)id partner transactions
- sig
string
(required)signature
API Withdraw ¶
If you have already integrated withdrawal to Geo account or payment of invoices, then withdrawal to the card can be done in the same way,
replacing the field receiver_hash_id
withcard_number
If in Respons you received a status of 500 or another error that is not described in the documentation, this does not mean that the payment did not go through. Wait and make a request for the status of your payment
Withdraw funds to credit card Visa/MasterCard ¶
Headers
Content-Type: application/json
Body
{
"data": "{
"api_key": "APIKEY0367c1943...eaf54",
"equivalent": "uahg",
"amount": "100",
"card_number": "1111222233334444",
"partner_transaction_id": "555bb23...ade3"
}",
"sig": "01fc...61e"
}
Headers
Content-Type: application/json
Body
{
"code": 0,
"message": "OK",
"data": "{
"transaction_uuid": "654srg...56dfg",
"partner_transaction_id": "555bb23...ade3",
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 8,
"message": "Payment system error.",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 20,
"message": "Equivalent not found.",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 1,
"message": "Some parameters are absent, or invalid.",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 3,
"message": "Amount is greater than ... max transaction amount.",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 22,
"message": "Duplicate partner_transaction_id.",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 13,
"message": "IP address not registered",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"data": "{
"api_key": "APIKEY0367c1943...eaf54",
"equivalent": "uahg",
"amount": "100",
"iban": "UA213223130000026007233566001",
"account_name": "Lastname Firstname Patronymic",
"account_number": "0123456789",
"partner_transaction_id": "555bb23...ade3"
}",
"sig": "01fc...61e"
}
Headers
Content-Type: application/json
Body
{
"code": 0,
"message": "OK",
"data": "{
"transaction_uuid": "654srg...56dfg",
"partner_transaction_id": "555bb23...ade3",
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 8,
"message": "Payment system error.",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 20,
"message": "Equivalent not found.",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 1,
"message": "Some parameters are absent, or invalid.",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 3,
"message": "Amount is greater than ... max transaction amount.",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 22,
"message": "Duplicate partner_transaction_id.",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 13,
"message": "IP address not registered",
"data": "{
"datetime": '"2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Withdraw funds to credit card Visa/MasterCardPOST/payment-systems/withdraw/
IMPORTANT! data
field is passed not as an object, but as a JSON string
- data
string
(required)JSON string
- api_key
string
(required)API KEY
- equivalent
string
(required)uahg, inrg, usdt
- amount
string
(required)amount
- card_number
string
(required)card number
- Required unless the following fields are provided:
- iban
- account_name
- account_number
- Required unless the following fields are provided:
- iban
string
(optional)IBAN
- Required only if card_number is not provided
- account_name
string
(optional)Account holder name
- Required only if card_number is not provided
- account_number
string
(optional)Taxpayer Identification Number
- Required only if card_number is not provided
- partner_transaction_id
string
(required)id partner transactions
- sig
string
(required)id partner transactions
WITHDRAWAL STATUS ¶
Headers
Content-Type: application/json
Body
{
"data": "{
"api_key":"APIKEY0367c1943...eaf54",
"transaction_uuid":"654srg...56dfg",
"partner_transaction_id": "555bb23...ade3"
}",
"sig": "00974e2.....3011"
}
Headers
Content-Type: application/json
Body
{
"code": 0,
"message": "OK",
"data": "{
"payment_system_name": "OneTwoPay",
"status": "PAID",
"start_date": "2020-03-11T12:05:13.223397+00:00",
"finish_date": "2020-03-11T12:07:24.223397+00:00",
"amount": "10",
"processed_amount": "10",
"withdraw_amount": "10.08",
"datetime": "2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
"status" - withdrawal status
Possible withdrawal statuses:
PENDING - processing
PAID - successfully paid
REJECTED, NODES_HANDLER_REJECTED or
REJECT - rejected, funds were not withdrawn
DONE_PARTIALLY - part of the funds was withdrawn to the card
"payment_system_name" - name of the payment system that processed the withdrawal
"start_date" - withdrawal creation date
"finish_date" - withdrawal completion date, may be null
"amount" - this amount will be credited to the card
"processed_amount" - this amount credited to the card. May be null, in which case the amount credited to the card is equal to "amount"
"withdraw_amount" - this amount will be debited from your GEO Pay account
Headers
Content-Type: application/json
Body
{
"code": 1,
"message": "Some parameters are absent, or invalid.",
"data": "{
"datetime": "2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 23,
"message": "Invalid transaction_id: request must contain only 1 parameter partner_transaction_id or transaction_uuid",
"data": "{
"datetime": "2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 23,
"message": "Invalid transaction_id: partner_transaction_id and transaction_uuid is None",
"data": "{
"datetime": "2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 13,
"message": "IP address not registered",
"data": "{
"datetime": "2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 2,
"message": "Withdrawal not found.",
"data": "{
"datetime": "2020-03-11T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Check withdrawal statusPOST/payment-systems/withdraw/status/
Parameters transaction_uuid
and partner_transaction_id
are optionals
To obtain a status, any one can be specified, but one of them must be specified.
IMPORTANT! data
field is passed not as an object, but as a JSON string
- data
string
(required)JSON string
- api_key
string
(required)API KEY
- transaction_uuid
string
(optional)id transactions GEO pay
- partner_transaction_id
string
(optional)id partner transactions
- sig
string
(required)signature
API Users ¶
Users. Get current user's balance ¶
Headers
Content-Type: application/json
Body
{
"data": "{
"api_key": "APIKEY0367c1943...eaf54",
"equivalent": "ushg"
}",
"sig": "01fc...61e"
}
Headers
Content-Type: application/json
Body
{
"code": 0,
"message": "OK",
"data": "{
"balance": 100,
"equivalent": "uahg",
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 1,
"message": "Some parameters are absent, or invalid.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 13,
"message": "Signature verification error.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 10,
"message": "Nodes handler respond with unknown code.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Get current user's balancePOST/account/user/balance/
IMPORTANT! data
field is passed not as an object, but as a JSON string
- data
string
(required)JSON string
- api_key
string
(required)API KEY
- equivalent
string
(required)uahg, kztg, inrg
- sig
string
(required)signature
Users. User data ¶
Headers
Content-Type: application/json
Body
{
"data": "{
"api_key": "APIKEY0367c1943...eaf54",
"hash_id": "45dcdf0c99e0...01d533f93a8b445cf27"
}",
"sig": "01fc...61e"
}
Headers
Content-Type: application/json
Body
{
"code": 0,
"message": "OK",
"data": "{
"first_name": "John",
"last_name": "Doe",
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 7,
"message": "Hash id is invalid.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 7,
"message": "Something went wrong",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 7,
"message": "Signature verification error.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
User dataPOST/applicant/info/
IMPORTANT! data
field is passed not as an object, but as a JSON string
To use this endpoint, you must obtain the appropriate permissions. Otherwise, the endpoint will not work! To obtain permission, contact your manager!
- data
string
(required)JSON string
- api_key
string
(required)API KEY
- hash_id
string
(required)id пользователя GEO pay
- sig
string
(required)signature
Payment history ¶
Payment history ¶
Headers
Content-Type: application/json
Body
{
"data": "{
"api_key": "APIKEY0367c1943...eaf54",
"start": "10",
"end": "50",
"datetime_from": "2023-03-10 00:00:00",
"datetime_to": "2023-03-11 00:00:00",
"equivalent": "uahg"
}",
"sig": "01fc...61e"
}
Headers
Content-Type: application/json
Body
{
"code": 0,
"message": "OK",
"data": "{
"transactions":[
{
"uuid": "abcabc1f-3333-2222-1111-0f2df6e6a2ed",
"datetime": "2023-02-08T12:58:18.223397+00:00",
"status": "DONE",
"amount": "1250",
"details": "Test payment",
"partner_transaction_id": "pay_1Q3k8g31",
"type": "DEPOSIT"
}",
{
"uuid": "abcab1q-4444-5555-6666-0sdgfd8sdf9sd",
"datetime": "2023-02-08T16:53:02.223397+00:00",
"status": "REJECTED",
"amount": "350",
"details": "Test payment 2",
"partner_transaction_id": "pay_2ew2lkj5",
"type": "WITHDRAWAL"
}",
{
"uuid": "abca3eq-5555-6666-7777-afdasf8sdf9sd",
"datetime": "2023-03-02T12:33:41.223397+00:00",
"status": "EXPIRED",
"amount": "900",
"details": "Test payment 3",
"partner_transaction_id": "pay_3432rkj5",
"type": "DEPOSIT"
}
]
}"
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 1,
"message": "Some parameters are absent, or invalid.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 13,
"message": "Signature verification error.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Headers
Content-Type: application/json
Body
{
"code": 10,
"message": "Nodes handler respond with unknown code.",
"data": "{
"datetime": "2019-07-08T12:58:18.223397+00:00"
}",
"sig": "0049d...2664a"
}
Get payment historyPOST/account/transactions/
IMPORTANT! data
field is passed not as an object, but as a JSON string
- data
string
(required)JSON string
- api_key
string
(required)API KEY
- start
int
(required)pagination of payments from
- end
int
(required)pagination of payments to
- datetime_from
string
(required)date/time from (isoformat)
- datetime_to
string
(required)date/time to (isoformat)
- equivalent
string
(required)uahg, kztg, inrg
- sig
string
(required)signature
Generated by aglio on 25 Sep 2024